You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
When attempting to create new models that contain multiple belongsTo annotations, it is returning the error 'Variable 'input' has coerced Null value for NonNull type 'ID!'
The code used to create the models and attempt to save in the database:
Friendship newFriendRequest = Friendship(
receiverID: receivingUser.id,
senderID: currentUser.id,
status: FriendStatus.Pending);
Friend sender = Friend(friendship: newFriendRequest, user: currentUser);
Friend receiver = Friend(friendship: newFriendRequest, user: receivingUser);
newFriendRequest = newFriendRequest.copyWith(users: [sender, receiver]);
Friendship? savedFriendRequest = await saveNewFriendship(newFriendRequest);
Friend? savedSender = await saveNewFriend(sender);
Friend? savedReceiver = await saveNewFriend(receiver);
Future<Friend?> saveNewFriend(Friend friendToSave) async {
try {
final request = ModelMutations.create(friendToSave); // LINE A
final response = await Amplify.API.mutate(request: request).response; // LINE B
if (response.errors.isNotEmpty) {
print('Could not save new friend: Response: ${response.errors}');
throw(response.errors);
}
return response.data;
} catch (error) {
print('Could not save new friend: ${error}');
Sentry.captureException(error, hint: 'Could not save new friend.');
}
return null;
}
Future<Friendship?> saveNewFriendship(Friendship newFriendship) async {
try {
final request = ModelMutations.create(newFriendship);
final response = await Amplify.API.mutate(request: request).response;
if (response.errors.isNotEmpty) {
print('Could not save new friendship: Response: ${response.errors}');
throw(response.errors);
}
return response.data;
} catch (error) {
print('Could not save new friendship: ${error}');
Sentry.captureException(error, hint: 'Could not save new friendship.');
}
return null;
}
The saving of the Friendship is successful, however, for each of the saving of the friends, the response returns a GraphQL error stating: 'Variable 'input' has coerced Null value for NonNull type 'ID!'
The reason I believe it has to do with the multiple belongsTo annotations is due to the ordering of the Friend model. The model coming into the saveNewFriend function appears correctly (Notated above as 'LINE A'), meaning it has all of the fields populated and the correct relationship. (See screenshots below). However, once the request object is returned from the ModelMutations.create() function, it DROPS the friendshipID (also in screenshots below). If I go to the schema.graphql file and SWAP the positions of the user/userID field with the friendship/friendshipID field, then the request object returned will DROP the userID resulting in the same error.
According to the documentation found here, it specifies that multiple belongsTo annotations should be allowed.
Categories
Analytics
API (REST)
API (GraphQL)
Auth
Authenticator
DataStore
Storage
Steps to Reproduce
Copy the models above in an Amplify Flutter project with API (GraphQL)
Create the models in the dart code.
Save the Friendship first.
Attempt to Save the Friend objects.
(Error should occur stating that the coerced Null value for ID!)
Screenshots
The friendToSave object being passed into the saveNewFriend method:
Above, you can see that both the User field and the Friendship field is populated in the Friend object.
The request object from 'LINE A' above:
After going through the ModelMutation.create() function, it drops the friendshipID field and the only inputs remaining are the ID from the Friendship, and the ID of the user field.
Here's the response of the call followed by the error:
Platforms
iOS
Android
Web
macOS
Windows
Linux
Android Device/Emulator API Level
API 32+
Environment
Doctor summary (to see all details, run flutter doctor -v):
[✓] Flutter (Channel stable, 3.0.5, on macOS 12.5 21G72 darwin-x64, locale en-US)
[✓] Android toolchain - develop for Android devices (Android SDK version 30.0.2)
[✓] Xcode - develop for iOS and macOS (Xcode 13.4.1)
[✓] Chrome - develop for the web
[✓] Android Studio (version 2021.2)
[✓] IntelliJ IDEA Community Edition (version 2018.3.3)
[✓] VS Code (version 1.69.2)
[✓] Connected device (4 available)
[✓] HTTP Host Availability
Thanks for the reply @haverchuck . The issue does seem similar. The only piece that I'm not sure about is that the issue you linked has no mention of the varying 'ID!' fields being dropped from the ModelMutations.create() function. This could be semantics, I just want to make sure that the other ticket notes that it's impossible to create records that contain the multiple 'belongsTo' annotated fields.
Description
When attempting to create new models that contain multiple belongsTo annotations, it is returning the error 'Variable 'input' has coerced Null value for NonNull type 'ID!'
Here are the model schemas being used:
The code used to create the models and attempt to save in the database:
The saving of the Friendship is successful, however, for each of the saving of the friends, the response returns a GraphQL error stating: 'Variable 'input' has coerced Null value for NonNull type 'ID!'
The reason I believe it has to do with the multiple belongsTo annotations is due to the ordering of the Friend model. The model coming into the saveNewFriend function appears correctly (Notated above as 'LINE A'), meaning it has all of the fields populated and the correct relationship. (See screenshots below). However, once the request object is returned from the ModelMutations.create() function, it DROPS the friendshipID (also in screenshots below). If I go to the schema.graphql file and SWAP the positions of the user/userID field with the friendship/friendshipID field, then the request object returned will DROP the userID resulting in the same error.
According to the documentation found here, it specifies that multiple belongsTo annotations should be allowed.
Categories
Steps to Reproduce
Screenshots
The friendToSave object being passed into the saveNewFriend method:
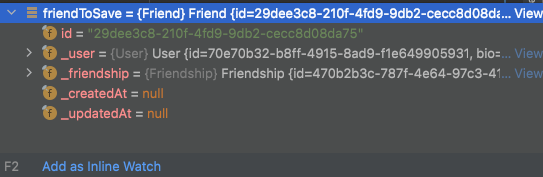
Above, you can see that both the User field and the Friendship field is populated in the Friend object.
The request object from 'LINE A' above:
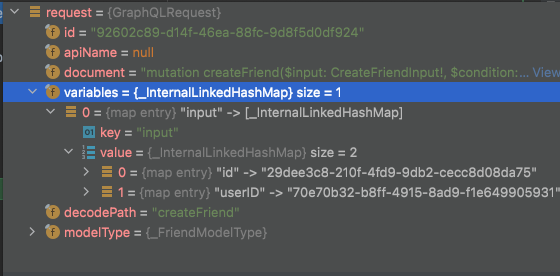
After going through the
ModelMutation.create()
function, it drops the friendshipID field and the only inputs remaining are the ID from the Friendship, and the ID of the user field.Here's the response of the call followed by the error:
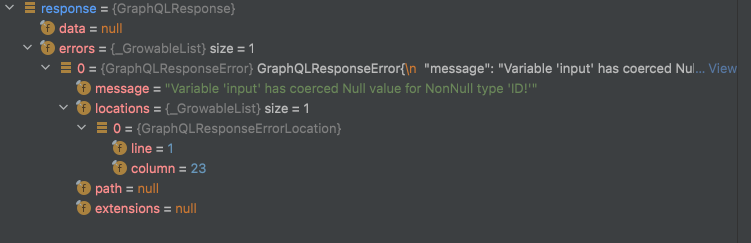
Platforms
Android Device/Emulator API Level
API 32+
Environment
Dependencies
Device
N/A
OS
N/A
Deployment Method
Amplify CLI
CLI Version
9.2.1
Additional Context
No response
Amplify Config
{
"UserAgent": "aws-amplify-cli/2.0",
"Version": "1.0",
"api": {
"plugins": {
"awsAPIPlugin": {
"": {
"endpointType": "GraphQL",
"endpoint": "",
"region": "us-east-2",
"authorizationType": "AMAZON_COGNITO_USER_POOLS"
}
}
}
},
"auth": {
"plugins": {
"awsCognitoAuthPlugin": {
"UserAgent": "aws-amplify-cli/0.1.0",
"Version": "0.1.0",
"IdentityManager": {
"Default": {}
},
"CredentialsProvider": {
"CognitoIdentity": {
"Default": {
"PoolId": "",
"Region": "us-east-2"
}
}
},
"CognitoUserPool": {
"Default": {
"PoolId": "<POOL_ID>",
"AppClientId": "<CLIENT_ID>",
"Region": "us-east-2"
}
},
"Auth": {
"Default": {
"OAuth": {
"WebDomain": "<WEB_DOMAIN>",
"AppClientId": "<APP_CLIENT_ID>",
"SignInRedirectURI": "",
"SignOutRedirectURI": "",
"Scopes": [
"phone",
"email",
"openid",
"profile",
"aws.cognito.signin.user.admin"
]
},
"authenticationFlowType": "USER_SRP_AUTH",
"socialProviders": [
"FACEBOOK",
"GOOGLE"
],
"usernameAttributes": [
"EMAIL"
],
"signupAttributes": [
"EMAIL",
"NAME"
],
"passwordProtectionSettings": {
"passwordPolicyMinLength": 8,
"passwordPolicyCharacters": []
},
"mfaConfiguration": "OPTIONAL",
"mfaTypes": [
"SMS"
],
"verificationMechanisms": [
"EMAIL"
]
}
},
"AppSync": {
"Default": {
"ApiUrl": "<APP_URI>",
"Region": "us-east-2",
"AuthMode": "AMAZON_COGNITO_USER_POOLS",
"ClientDatabasePrefix": "_AMAZON_COGNITO_USER_POOLS"
},
"_AWS_IAM": {
"ApiUrl": "<APP_URI>",
"Region": "us-east-2",
"AuthMode": "AWS_IAM",
"ClientDatabasePrefix": "_AWS_IAM"
}
},
"S3TransferUtility": {
"Default": {
"Bucket": "",
"Region": "us-east-2"
}
}
}
}
},
"storage": {
"plugins": {
"awsS3StoragePlugin": {
"bucket": "",
"region": "us-east-2",
"defaultAccessLevel": "guest"
}
}
}
}
The text was updated successfully, but these errors were encountered: