-
-
Notifications
You must be signed in to change notification settings - Fork 25
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
perf(mspc): replace bad VecDeque wait queue with intrusive list (#16)
The new wait queue implementation doesn't allocate, and doesn't require resizing a `VecDeque` inside a lock. This should improve performance and (hopefully) make it possible to use the MPSC queue without any allocations on no-std (once I figure out the static stuff). Performance for blocking/yielding send is now very competitive after switching to the new wait queue, compare the new violin plot of the integer MPSC benchmark with violin plot with the old wait queue that i posted in #14. Old'n'busted: 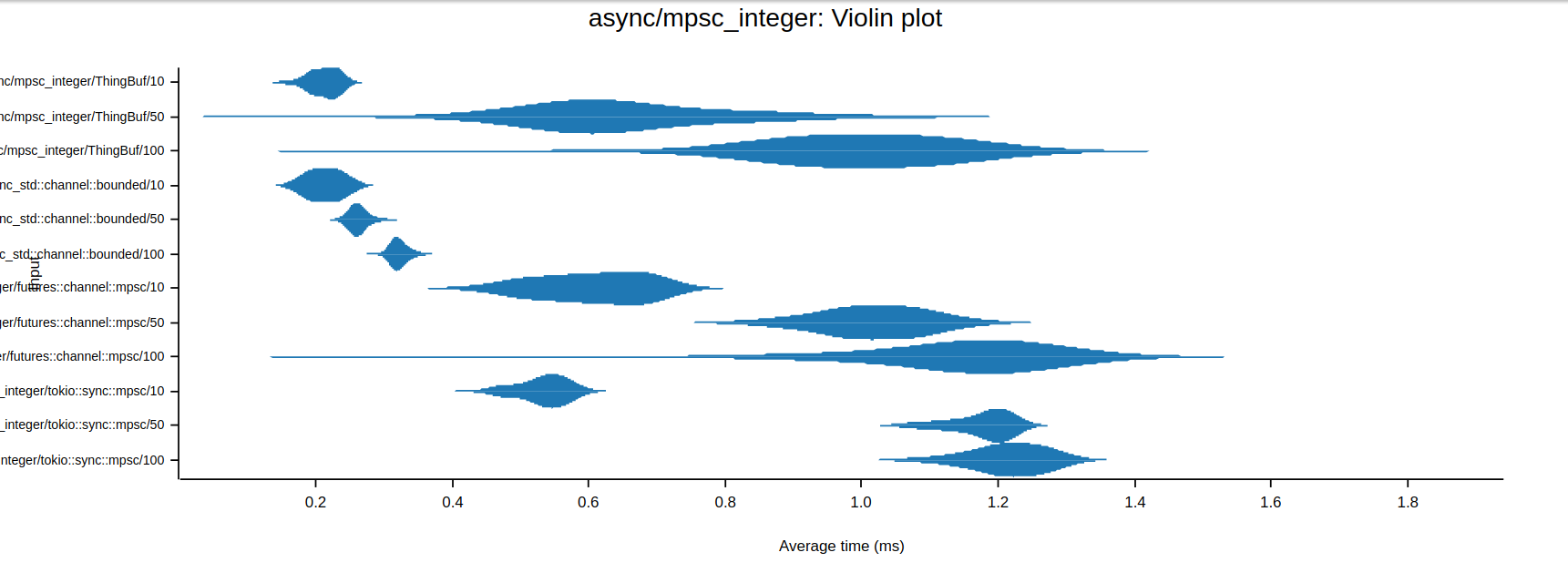 New hotness: 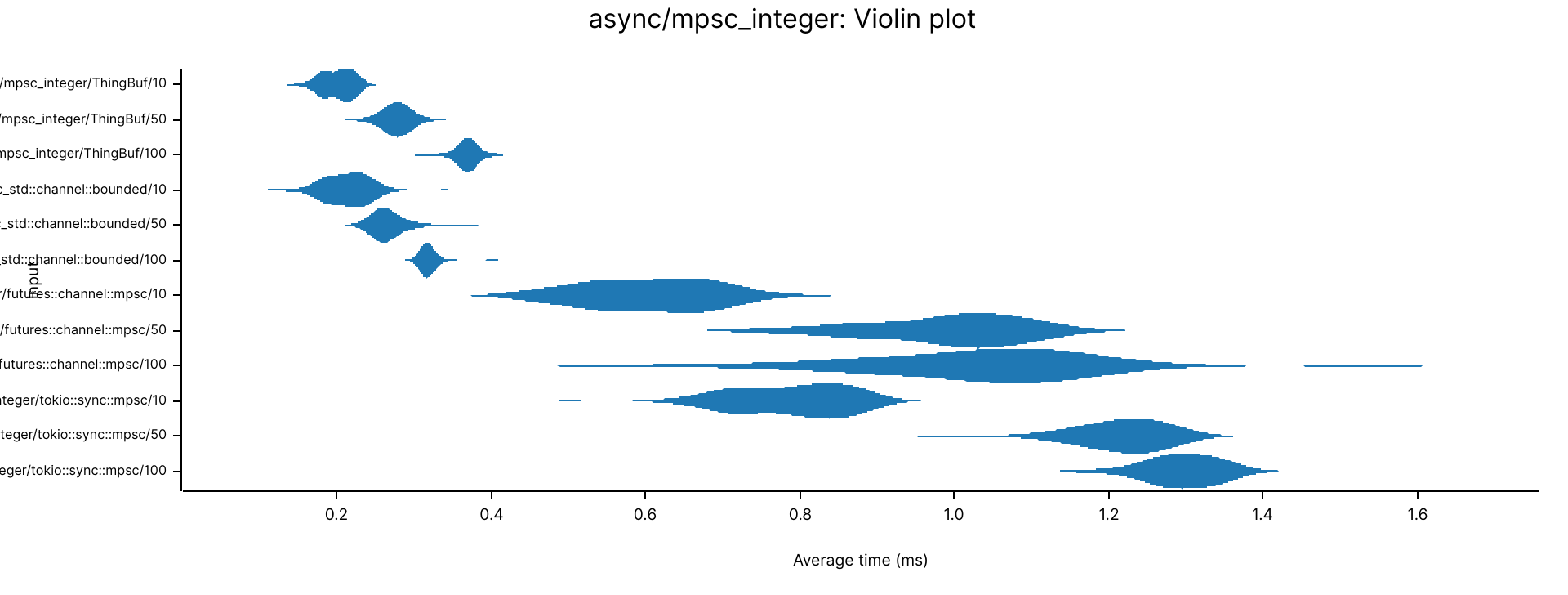 Big comparison benchmark: 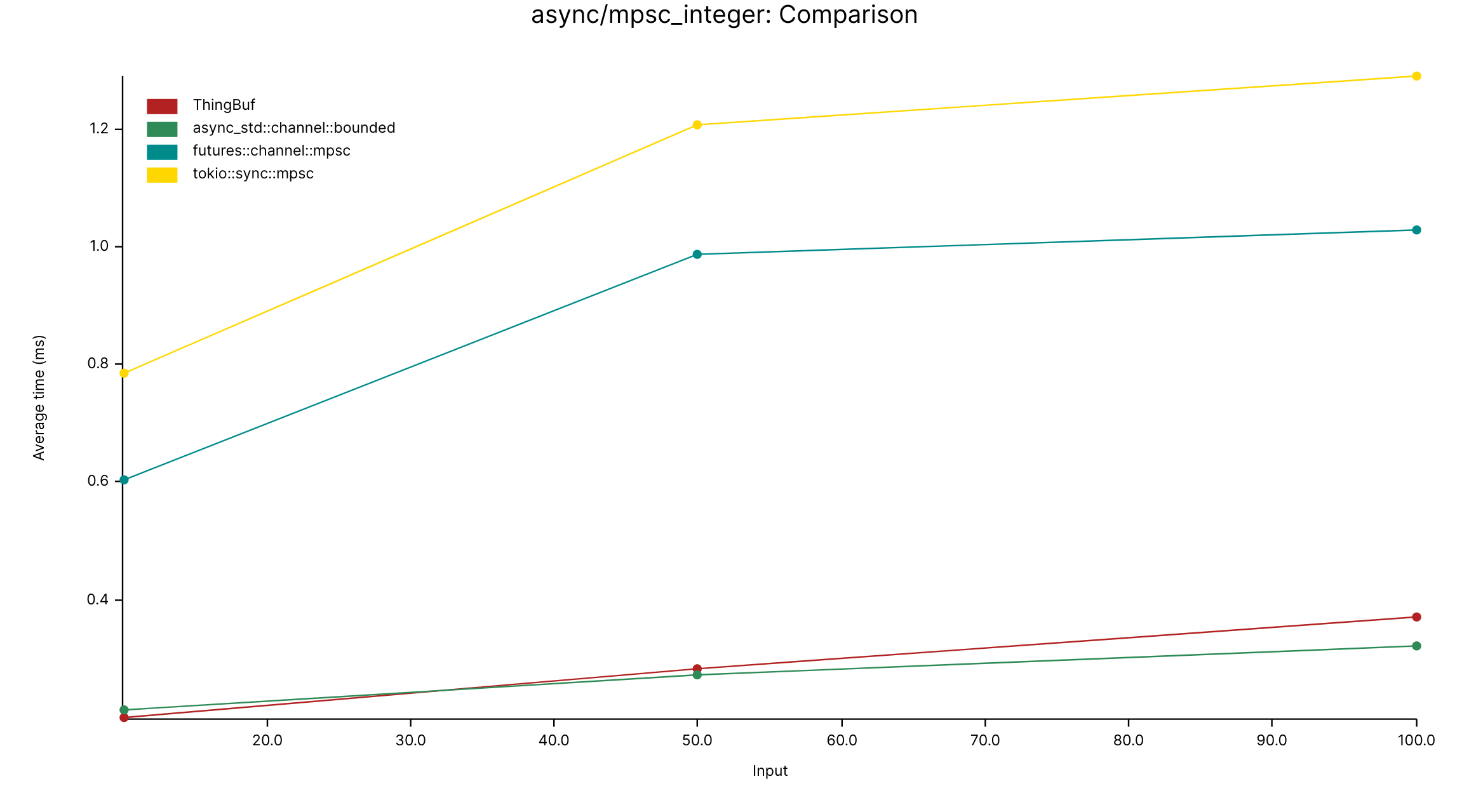 Signed-off-by: Eliza Weisman <[email protected]>
- Loading branch information
Showing
14 changed files
with
580 additions
and
319 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
feature! { | ||
#![feature = "std"] | ||
pub(crate) use self::std_impl::*; | ||
mod std_impl; | ||
} | ||
|
||
#[cfg(not(feature = "std"))] | ||
pub(crate) use self::spin_impl::*; | ||
|
||
#[cfg(any(not(feature = "std"), test))] | ||
mod spin_impl; |
Oops, something went wrong.