-
Notifications
You must be signed in to change notification settings - Fork 529
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Chore: Import only lodash submodules (#2041)
## Which problem is this PR solving? - resolves #2032 ## Description of the changes - Import only lodash submodules ## How was this change tested? - locally Super cool that it reduced the size taken by lodash bundle by 70.59% (master branch vs this) Before: 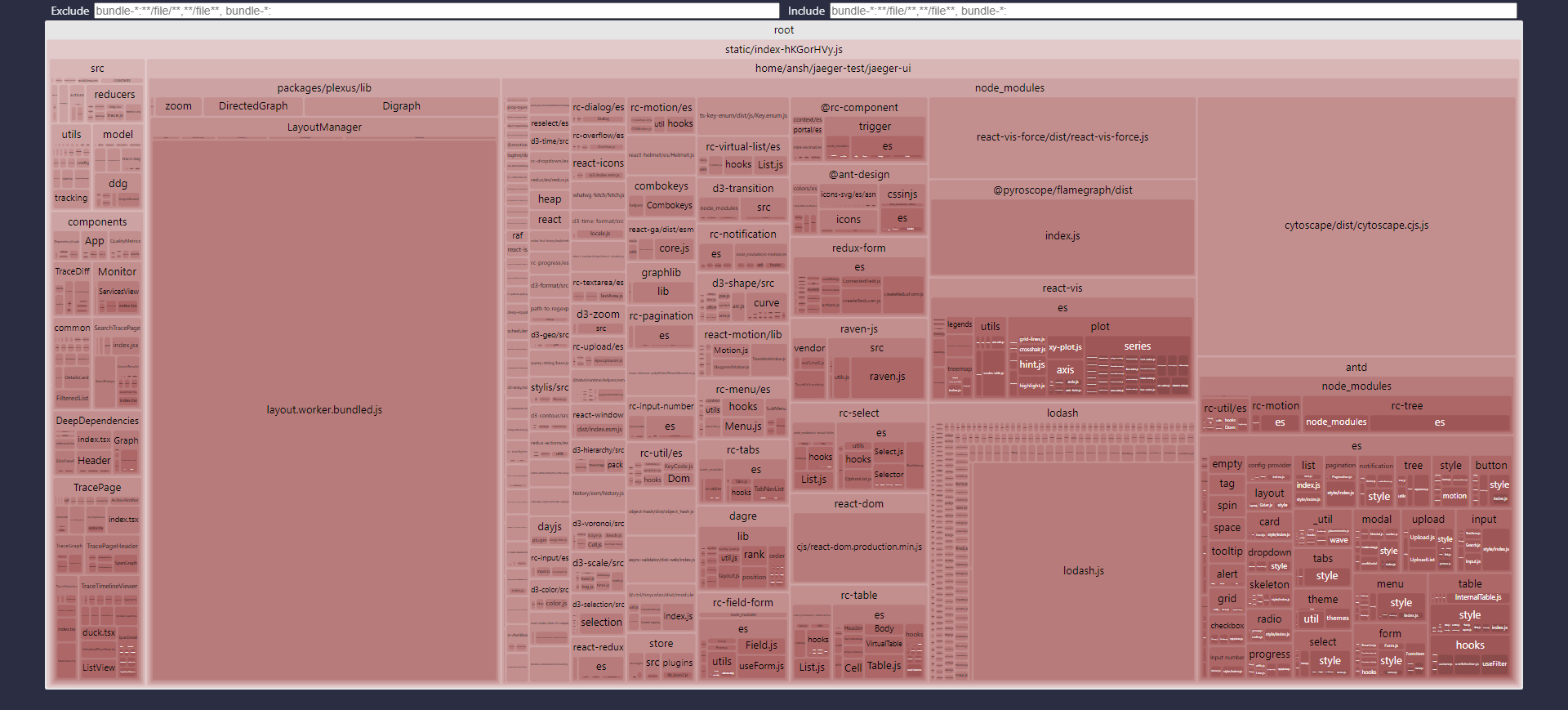 lodash: 773.55 KB After: 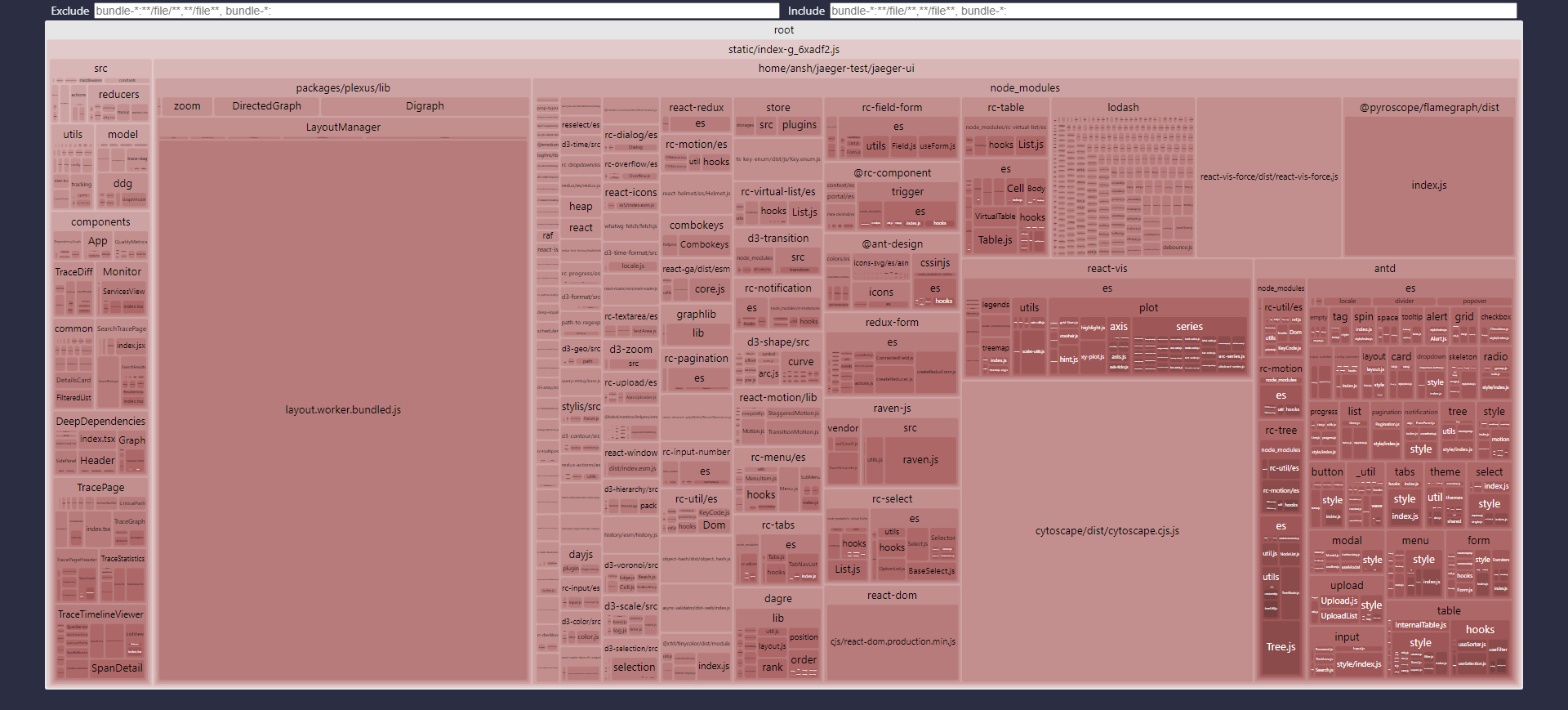 lodash: 227.43 KB ## Checklist - [x] I have read https://github.com/jaegertracing/jaeger/blob/master/CONTRIBUTING_GUIDELINES.md - [x] I have signed all commits - [x] I have added unit tests for the new functionality - [x] I have run lint and test steps successfully - for `jaeger`: `make lint test` - for `jaeger-ui`: `yarn lint` and `yarn test` --------- Signed-off-by: Ansh Goyal <[email protected]>
- Loading branch information
1 parent
4bdb8ac
commit 46f89af
Showing
2 changed files
with
21 additions
and
42 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters