-
Notifications
You must be signed in to change notification settings - Fork 12.6k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Regression with >= v3 and React, generics, defaultProps #26395
Comments
The "regression" is due to TypeScript 3.0 adding support for the class Component<P, S> {
readonly props: Readonly<{ children?: ReactNode }> & Readonly<P>;
// ...
}
type LibraryManagedAttributes<C, P> =
C extends { propTypes: infer T; defaultProps: infer D; }
? Defaultize<MergePropTypes<P, PropTypes.InferProps<T>>, D>
: C extends { propTypes: infer T; }
? MergePropTypes<P, PropTypes.InferProps<T>>
: C extends { defaultProps: infer D; }
? Defaultize<P, D> // <--- This case applies.
: P;
type Defaultize<P, D> = P extends any
? string extends keyof P ? P :
& Pick<P, Exclude<keyof P, keyof D>>
& Partial<Pick<P, Extract<keyof P, keyof D>>>
& Partial<Pick<D, Exclude<keyof D, keyof P>>>
: never; The expected type of the attributes is based on the JSX.LibraryManagedAttributes<typeof FieldFeedback,
Readonly<{ children?: ReactNode }> & Readonly<P>> The important things here are the interface Props {
when: (value: string) => boolean;
}
function bad<P extends Props>(
attrs: string extends keyof P ? {[K in keyof P]: P[K]} : {[K in keyof P]: P[K]}) {}
function good1<P extends Props>(
attrs: string extends keyof P ? P : {[K in keyof P]: P[K]}) {}
function good2<P extends Props>(
attrs: {[K in keyof P]: P[K]}) {}
bad({when: value => false});
good1({when: value => false});
good2({when: value => false}); Before using the type of the I hope this partial analysis is helpful. I don't have a good enough understanding of the intended purposes of the compiler concepts to go any further in speculating about what would be an appropriate fix. |
I opened #26281 as a partial fix for this when I saw this appear in our user suite btw. It at least makes generic index types simplifiable much more often. |
@mattmccutchen I can confirm that your reduced example now builds correctly in |
The original project belonged to @tkrotoff... |
ah, doh. @tkrotoff I mean. 😺 |
ahhh, looking at @tkrotoff 's example, the full |
Righto, so another problem with Two things. |
Scratch that. Has nothing to do with any of that. Real remaining issue is that the contextual type created by inference isn't being applied to the attributes correctly. Haaaaaaaaaaaaaaaaaah. |
Both of your posts actually seriously helped expedite fixing this, so thanks to y'all. Problems which arise in JSX are often complex with many moving parts within the compiler, so reduced repros really help. |
Interesting. React's types are ridiculously complicated, so it's very possible there's multiple layers of edge cases or bugs working against you here. I'll certainly try to look into it.
Actually directly my fault, sorry. The fix for that (we found it in the nightlies) didn't quite make the 2.9 release cycle and got bumped to 3.0. 😦 It happens sometimes.
I just opened a PR to fix that, thanks to your comment telling me it existed. Sorry bud, sometimes issues haven't been quite triaged correctly right now, since we just cycled to a new manager here and some stuff may have not been triaged correctly during the transition (we get a lot of issue activity - notifications on this repo are almost impossible to use). That one should definitely have been put into 3.1, which we still haven't made a final build for, so hopefully it'll be fixed in the final 3.1 release.
Since we didn't end up merging #21070 we're waiting on something like #26797 to lay the groundwork for making it easier to remove some simplifying assumptions we have in place regarding computed property names of instantiable types and unions. It's certainly a bit inconvenient for now, but we have a lot of concerns to work through for any given feature; so it can take awhile as we design and iterate.
You've written async resetFields(...inputsOrNames: Array<TextInput | string>); right above your async resetFields(...inputsOrNames: Array<TextInput | string>) {}; // Note the curly braces! or write it as an optional property if the intent is for it not to be implemented but still be in a concrete (not resetFields?: (...inputsOrNames: Array<TextInput | string>) => Promise<void>; or add an export abstract class FormWithConstraints extends _FormWithConstraints {
//...
abstract async resetFields(...inputsOrNames: Array<TextInput | string>): void; // Note I also included a return type annotation. You should probably do that, too.
// ...
} |
Got it. I actually had a version of my original fix that fixed it fully but backed down from it because it didn't seem necessary on second glance (and was a bit inelegant). Turns out it was. #27251 is open to fix. |
I encountered a regression with TypeScript >= 3 + React, generics and defaultProps
TypeScript versions: 3.1.0-dev.20180810 and 3.0.1, not with < 3
Search terms: React defaultProps generics
Code:
Expected behavior: No error
Actual behavior:
Parameter 'value' implicitly has an 'any' type
at linewhen={value => ...}
When removing
defaultProps
, there is no error:Error vscode (using 3.1.0-dev.20180810) screenshot:
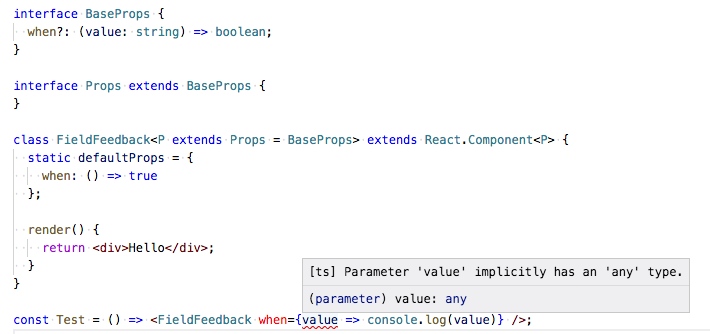
No error vscode (using 3.1.0-dev.20180810) screenshot:
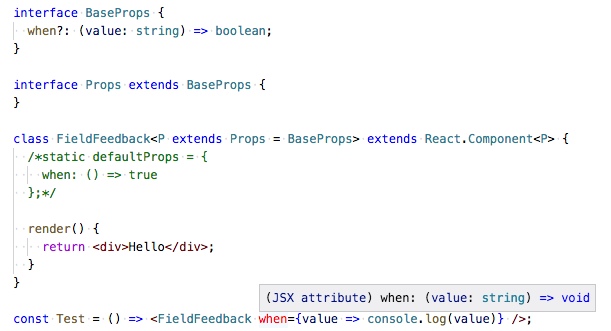
Stumble upon this while upgrading this lib: https://github.com/tkrotoff/react-form-with-constraints to TypeScript 3.
I do not think this is related with @types/react. Using v16.4.9 (latest version) it fails with TypeScript >= 3 and works with TypeScript < 3:
The text was updated successfully, but these errors were encountered: